Source Maps
Overview
Source Maps help to convert minified JavaScript code back into source code. Raygun uses them to take un-readable errors generated from minified JavaScript and translate them to be readable and to include code snippets from your source.
For a more in-depth explanation have a look at our Source maps blogpost
How to upload source maps
There are three main ways to upload your source maps to Raygun
1. Automatically
If your source maps are publicly available on your website Raygun will fetch them automatically without you having to do anything. If you do not want your source maps to be public but still want Raygun to be able to automatically fetch them you can setup an auth key in the JS source map center that will be sent by Raygun when requesting source maps that you can validate on your server
2. Manually
If the automatic option does not work for your application you can also manually upload source maps either through our website using the JS source map center
or via our API
Uploading using the JS source map center
You can find the JS source map center through the application settings
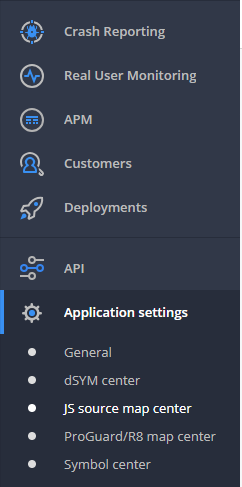
At the bottom of the page you can see a list of all of your current source maps and a file picker for uploading new ones. After uploading a source map it is important to add the correct URI in the URL field for the source map, if this is not set correctly then Raygun will fail to use your source map when attempting to desymbolicate a stack trace
Uploading using the API
Uploading a source map using Raygun' API requires three pieces of information in addition to the source map file
For more information about the source map upload endpoint and other source map endpoints please refer to the Raygun API docs.
1. A Personal access token token You can generate a PAT token in your user settings
2. Your Raygun application identifier You can find the your application identifier (as well as the full API URL) in the JS source map center
3. The URI to the minified file This is the URI that the source maps corresponding JavaScript file can be accessed on your website or application. If you are unsure what your source maps corresponding URI is see the Finding a source maps URI section
To upload a source map, you need to provide a multipart/form-data request with the following fields:
- file (required): The source map file you wish to upload. This file must be sent as binary data.
- uri (required): The URI associated with the source map file. This must be a valid URI format.
Example of a curl
request:
curl -X POST 'https://api.raygun.com/v3/applications/{your-application-identifier}/source-maps' \
-H 'Authorization: Bearer YOUR_PERSONAL_ACCESS_TOKEN' \
-H 'Content-Type: multipart/form-data' \
-F 'file=@path_to_your_source_map_file.map' \
-F 'uri=your_source_map_uri'
3. Using build plugins
Raygun offers plugins for popular JavaScript bundlers that will attempt to automatically collect and upload source maps when you build your site.
Currently only a Webpack plugin is available, if you have suggestions for an additional one or perhaps want to develop one yourself please open an issue or pull request on the GitHub repository Plugins
Mobile apps
With many mobile applications being written in JavaScript, using Raygun4js is an appealing way to track errors across all platforms. However, as the application is hosted on the phone instead of a website with a static URL you may find that error stack traces contain files paths which differ across different platforms and devices. This introduces some difficulty when trying to utilize source maps which require the minified and mapping files to be uploaded and labeled with exactly the same URL's as would appear in a stack trace.
For this reason we recommend adding a handler to the raygun4JS provider to rewrite file's URLs before the error is reported back to Raygun. This means that errors will be reported from the same domains and not unique domains for every platform or device.
An example of such a handler is shown below:
//handler method
var beforeSend = function(payload) {
var stacktrace = payload.Details.Error.StackTrace;
var normalizeFilename = function(filename) {
var indexOfJsRoot = filename.indexOf("js");
return 'https://normalizedurl.com/' + filename.substring(indexOfJsRoot);
}
for(var i = 0 ; i < stacktrace.length; i++) {
var stackline = stacktrace[i];
stackline.FileName = normalizeFilename(stackline.FileName);
}
return payload;
}
//attaching the handler to the Raygun provider
Raygun.onBeforeSend(beforeSend);
This handler removes the platform and device specific paths from the URL's present in an error's stacktrace.
For instance an error occurring on an Android phone within the file:
file://android_asset/www/js/myjsfile.min.js
and on an iOS device within the file:
file://accounts/1234/appdata/b12b33f1-519b-4d1c-8d68-315513ecbac1/www/js/myjsfile.min.js
will both report:
https://normalizedurl.com/js/myjsfile.min.js
as the URL of the file within which the error occurred.
This allows the use of a single set of source maps for source mapping across all deployments.
Notes
There could be up to a 30-minute delay before the mapping process starts utilizing any uploaded files. To reprocess any errors which occur during this time simply hit the "Re-process this error for Source Maps" button on an error instance.
Code snippet insertion is only available if there are less than 50 source files referenced in the map file.